Features
Key Value Storage
Add a global, low-latency key-value data storage to your Nuxt application.
Getting Started
Enable the key-value storage in your NuxtHub project by adding the kv
property to the hub
object in your nuxt.config.ts
file.
nuxt.config.ts
export default defineNuxtConfig({
hub: {
kv: true
}
})
This option will use Cloudflare platform proxy in development and automatically create a Cloudflare Workers KV namespace for your project when you deploy it.
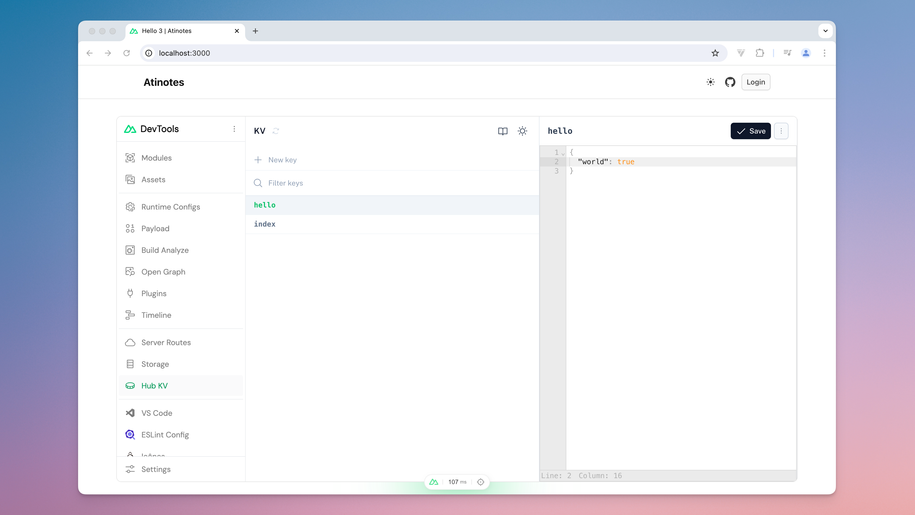
hubKV()
Server method that returns an unstorage instance with keys()
, get()
, set()
and del()
aliases.
keys()
Retrieves all keys from the KV storage (alias of getKeys()
).
const keys = await hubKV().keys()
/*
[
'react',
'react:gatsby',
'react:next',
'vue',
'vue:nuxt',
'vue:quasar'
]
To get the keys starting with a specific prefix, you can pass the prefix as an argument.
const vueKeys = await hubKV().keys('vue')
/*
[
'vue:nuxt',
'vue:quasar'
]
*/
get()
Retrieves an item from the Key-Value storage (alias of getItem()
).
const vue = await hubKV().get('vue')
/*
{
year: 2014
}
*/
set()
Puts an item in the storage (alias of setItem()
)
await hubKV().set('vue', { year: 2014 })
You can delimit the key with a :
to create a namespace:
await hubKV().set('vue:nuxt', { year: 2016 })
has()
Checks if an item exists in the storage (alias of hasItem()
)
const hasAngular = await hubKV().has('angular')
del()
Delete an item from the storage (alias of removeItem()
)
await hubKV().del('react')
...()
You can use any other method from unstorage as well.